How To Add Something To A Set In Python
In this tutorial, you'll larn how to append a value to a set up in Python using the .add together()
and .update()
methods. You lot'll learn a brief overview of what Python sets are. And then, you'll learn how to add a unmarried detail to a set using the .add()
method as well as multiple items using the .update()
method. You'll too learn how to utilize the chain operator to add items to a gear up.
Quick Answer: Use add
and update
to Append Items to a Set
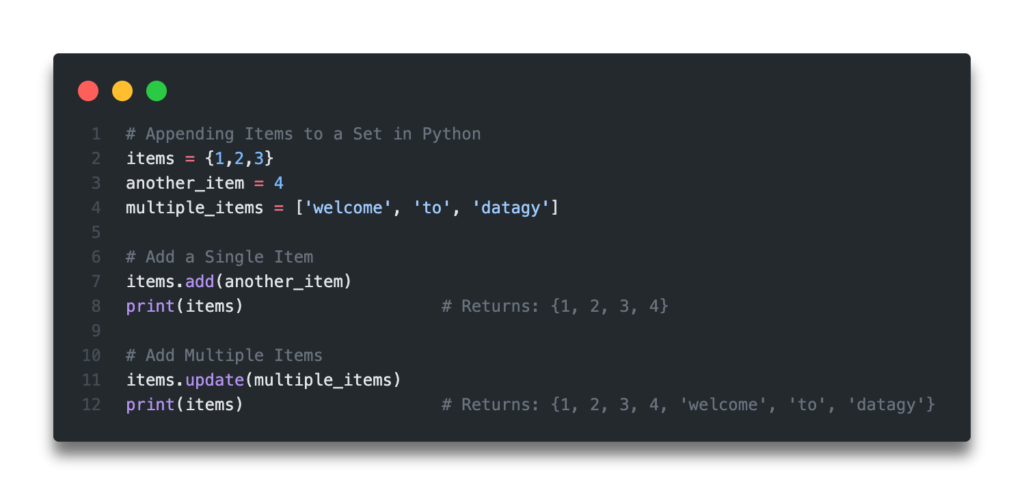
What are Python Sets?
Python sets are a information collections that are like to, say, Python lists and Python dictionaries. They do have some unique attributes: namely, Python sets are
- Unordered, meaning that you can't index a fix
- Contain only unique items, meaning that there cannot be any duplicates in the set.
Sets are created using curly braces ({}
), which may seem similar to Python dictionaries. Because of the similar declaration, you need to use the set up()
part to create an empty set.
Let's look at how nosotros tin create a set in Python:
# Creating an Empty Fix a_set = {} # Creating a Ready with Data another_set = {1, 2, 3, 4}
Because Python sets are unordered, we can't just change an item using an index. We can, still, use set methods to add or remove items from a set. Allow's dive into how to add a single particular to a set.
Add an Item to a Python Fix using Add together
The easiest way to add together a single item to a Python set up is by using the Python set method, .add()
. The method acts on a set and takes a single parameter, the detail to add. The item being added is expected to be an immutable object, such as a string or a number.
Let'southward see what this await like:
# Append to a Python set with .add() items = {1, 2, iii} items.add together(4) print(items) # Returns: {one, 2, 3, 4}
We can see that the method works in identify, allowing us to add an item without needing to re-assign the set to itself.
Now, what happens when nosotros try to add together an item that already exists in the prepare?
# Appending an existing particular to a Python set with .add() items = {1, 2, 3} items.add(3) impress(items) # Returns: {1, two, iii}
Because Python sets can simply comprise unique items, when an item that already exists is appended to the gear up, the set remains unchanged.
Now, permit'south come across what happens when nosotros endeavor to add an iterable object, such as a list to our fix:
# Suspend to a Python set up with .add together() items = {1, 2, three} items.add([4, 5, 6]) print(items) # Returns: TypeError: unhashable type: 'list'
We can see that when we try to append a mutable object, such as a list, that a TypeError
is raised. This is because sets can only contain immutable data types, as they cannot exist allowed to be changed.
In many cases, you'll want to add together multiple items to a ready. That'southward exactly what you'll learn in the next section.
Add Multiple Items to a Python Fix using Update
The Python .add together()
method merely accepts a single item. What happens when you want to append multiple items to a gear up? While information technology's possible to write a for loop to suspend multiple items using the .add()
method, Python also provides the .update()
method that accepts an iterable object to suspend multiple items to a prepare.
Let'south see what this looks like:
# Appending Multiple Items to a Python Set items = {i, ii, 3} new_items = [four, five, six] # You could use a for loop with .add together() for item in new_items: items.add(particular) impress(items) # Returns: {one, ii, 3, 4, 5, 6} # Or you could use the .update() method items = {1, 2, 3} new_items = [iv, v, 6] items.update(new_items) print(items) # Returns: {i, 2, iii, 4, v, 6}
We can run across that the by using the .update()
adds multiple items easily to a set.
Similar to using the .add()
method, if nosotros endeavor to append items that already be, they will simply be ignored. We can ostend this by trying it out:
# Appending Multiple Items to a Python Set items = {1, ii, three} new_items = [2, three, 4, 5, 6] items.update(new_items) print(items) # Returns: {1, 2, three, 4, v, half-dozen}
In the next section, you'll acquire how strings can be appended to a Python prepare.
Adding Strings to Python Sets
An interesting annotation near appending strings to Python sets is that they can exist appended using both the .add()
and .update()
methods. Depending on which one you cull will yield different results. This is because Python strings are technically immutable, just iterable objects.
Because of this, when a string is added using the .add()
method, the cord is added as a single item. Still, when a string is added using the .update()
method, it's added as separate items from the string. Let'southward run into what this looks like:
# Appending a string to a set in Python items1 = {1, 2, 3} items2 = {1, ii, 3} word = 'datagy' items1.add(word) items2.update(give-and-take) print('items1 = ', items1) print('items2 = ', items2) # Returns: # items1 = {'datagy', 1, ii, iii} # items2 = {1, 2, iii, 'a', 'd', 't', '1000', 'y'}
A way that we can alter this behaviour is by passing in the string every bit a list into the .update()
method. This way, Python will interpret the string as an item in the list, non as the iterable object itself. Allow's confirm this:
# Appending a string to a set in Python items = {one, 2, 3} word = 'datagy' items.update([word]) impress(items) # Returns: {ane, 2, 3, 'datagy'}
In the next section, you'll learn how to use the Python chain operator to append to a gear up in Python.
Use the Concatenation Operator to Append to a Set
Python also comes with a chain operator, |=
, which allows us to easily concatenate items. We can combine ii sets together using this operator, which results in a set up that contains the items of the commencement and the 2nd set.
Let's see how this works by appending one set to another:
# Concatenating two sets in Python items = {ane, 2, three} more_items = {iii, 4, five} items |= more_items impress(items) # Returns: {ane, 2, 3, 4, 5}
Here, nosotros instantiated two sets. We and so applied the concatenation to i of the sets to append the other, in place.
Conclusion
In this tutorial, you learned how to append to a set in Python. You learned how to use the .add()
and .update()
methods to add an detail or multiple items to a set up. So, you learned the quirks of working with strings and how to properly append them to a set. Finally, you lot learned how the Python concatenation operator |=
works in appending one ready to another.
To learn more than about the Python .add()
and .update()
methods, check out the official documentation here.
Additional Resources
To learn more most related topics, check out these articles:
- Pandas: Count Unique Values in a GroupBy Object
- Python: Count Unique Values in a List (4 Means)
- Python: Count Number of Occurrences in List (6 Ways)
- Python: Remove Duplicates From a Listing (vii Ways)
Source: https://datagy.io/python-append-set/
0 Response to "How To Add Something To A Set In Python"
Post a Comment